I am trying to add a calculation that would calculate the loan amount based on various variables. The equation is P * ((J) / (1-J)^n) . There is not an exponential operator and I do not see at the moment how I would calculate this. Has anyone experienced a similar issue and how did you solve it?
Using a JavaScript trigger you should be able to calculate using your equation and push the results back into another variable.
Create variables for J, P, n, and output.
Create a JavaScript trigger to grab the values entered in for J, P, n and return the equation results in output.
var player=GetPlayer();
//grab the equation inputs from SL var J=player.GetVar("J"); var P=player.GetVar("P"); var n=player.GetVar("n"); //run the calculation and push into the SL variable called output results=P*(J/(Math.pow((1-J), n))); player.SetVar("output",results);
I thank goodness every day that we have a Steve Flowers in the community. I still don't understand this question, even after seeing your lovely answer, Steve.
I may have screwed up the equation in JS. Didn't test it. Careful arrangement of parens should get it.
When testing, you'll want to publish to web and test from a server, publish to CD, or test the HTML5 version of the output. Flash player blocks JavaScript calls by default when content is run locally.
Hey Steve, thanks a lot for your help on this. My final equation was:
//grab the equation inputs from SL var P=player.GetVar("LoanAmount"); var J=player.GetVar("InterestRate"); var n=player.GetVar("NumberOfMonths") //run the calculation and push into the SL variable called output //results=(Math.pow((1+J),-n)); results=P*(J/(1-(Math.pow((1+J),-n)))); player.SetVar("MonthlyPayment",results);
This is a great function and will allow a ton of flexibility from the base functions.
I copied the javascript code but could you please let me know how to show in the storyline the calculated variable output/results?
Please see the attachment, all variables are there, but the result is not shown. It keeps showing a zero value when I try to show: %output% or %results%
2 basic errors in your script you could have found yourself when using the browser console to test when publishing.
Publish for Web, open it up and open the Console. F12 or CTRL+Shift+I
You will notice the error... exeJavascript - player is not defined. That points to an error in somewhere in your script you call the Storyline player, but donot declare it.
As Storyline triggers all have their own scope...you need to make sure you have access to the player in every trigger. Global scope can be achieved but thats for another time.
So add this in your trigger: var player = GetPlayer();
After this change publishing again...you will notice it will not work. And you have another error. This one...
In fact a similar error as before, but now indicating that the variable N is not defined. Checking your code you immediately notice that there is a variable 'N' but it is not called. Instead in your script you call variable 'n'. Do take good care that in Javascript a single character can make a big difference. When calling variables all names need to be exactly the same. Make a habit of using a consequent way of naming your variables and calling them.
I use Camelcase coding. Meaning a variable is always written like this. mygoodVariable Or use always CAPITALS or only undercast. Whatever works for you...but keep it consistent. And use the console to check your code.
So changing your code to this...and all works. var N=player.GetVar("N");
10 Replies
Hi Michael,
What product are you using (Storyline or Presenter)?
I am using Storyline.
Using a JavaScript trigger you should be able to calculate using your equation and push the results back into another variable.
Create variables for J, P, n, and output.
Create a JavaScript trigger to grab the values entered in for J, P, n and return the equation results in output.
var player=GetPlayer();
//grab the equation inputs from SL
var J=player.GetVar("J");
var P=player.GetVar("P");
var n=player.GetVar("n");
//run the calculation and push into the SL variable called output
results=P*(J/(Math.pow((1-J), n)));
player.SetVar("output",results);
I thank goodness every day that we have a Steve Flowers in the community. I still don't understand this question, even after seeing your lovely answer, Steve.
Thanks Steve...I am going to try this today and will let everyone know the results.
Great! Couple of notes:
Hey Steve, thanks a lot for your help on this. My final equation was:
//grab the equation inputs from SL
var P=player.GetVar("LoanAmount");
var J=player.GetVar("InterestRate");
var n=player.GetVar("NumberOfMonths")
//run the calculation and push into the SL variable called output
//results=(Math.pow((1+J),-n));
results=P*(J/(1-(Math.pow((1+J),-n))));
player.SetVar("MonthlyPayment",results);
This is a great function and will allow a ton of flexibility from the base functions.
Thank you Steve and colleagues!
I copied the javascript code but could you please let me know how to show in the storyline the calculated variable output/results?
Please see the attachment, all variables are there, but the result is not shown. It keeps showing a zero value when I try to show: %output% or %results%
2 basic errors in your script you could have found yourself when using the browser console to test when publishing.
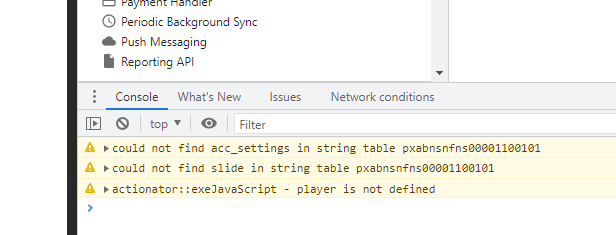
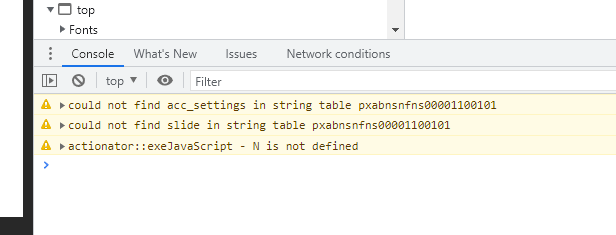
Publish for Web, open it up and open the Console. F12 or CTRL+Shift+I
You will notice the error... exeJavascript - player is not defined.
That points to an error in somewhere in your script you call the Storyline player, but donot declare it.
As Storyline triggers all have their own scope...you need to make sure you have access to the player in every trigger. Global scope can be achieved but thats for another time.
So add this in your trigger:
var player = GetPlayer();
After this change publishing again...you will notice it will not work.
And you have another error.
This one...
In fact a similar error as before, but now indicating that the variable N is not defined.
Checking your code you immediately notice that there is a variable 'N' but it is not called.
Instead in your script you call variable 'n'.
Do take good care that in Javascript a single character can make a big difference. When calling variables all names need to be exactly the same.
Make a habit of using a consequent way of naming your variables and calling them.
I use Camelcase coding. Meaning a variable is always written like this. mygoodVariable
Or use always CAPITALS or only undercast. Whatever works for you...but keep it consistent.
And use the console to check your code.
So changing your code to this...and all works.
var N=player.GetVar("N");
Thank you very much, Math!
Very useful and appreciated feedback!