I have a page with text entry fields. I want to disable / enable fields depending on the value of a variable. Is it possible to enable/disable text entry fields?
Bumping this thread to add another reason for disabling text entry fields: When reviewing a quiz question, a user can still tab through the focus order and change their answer, even with an object blocking the fields. Then when they return to the results slide it will mark that question as correct.
Thank you for your suggestion, but unfortunately this technique doesn't work when building a drop-down menu system that's embedded within a scroll panel. The layers are unable to track the location of the object within the scroll panel ... a layer simply shows up on top of whatever the existing content is.
I attached the .story file. The JavaScript code is inside Execute JavaScript triggers. Use it however and whenever you need. In this .story, I show how to simply enable or disable the text entry field with one button based on its state. In reply to Griffin Davis.
You should change selector querySelector('.acc-textinput') to querySelectorAll('.acc-textinput'). It selects all elements/inputs that match a specified CSS selector and returns a NodeList containing those elements. For example:
var inputs = document.querySelectorAll('.acc-textinput'); if (inputs.length > 0) { inputs[1].disabled = true; inputs[1].style.setProperty('color', '#BFBFBF', 'important'); }
It means that only the second element/input (input[0] being the first input) will be disabled. And enabled again with the code below:
var inputs = document.querySelectorAll('.acc-textinput'); if (inputs.length > 0) { inputs[1].disabled = false; inputs[1].style.setProperty('color', 'rgba(0, 0, 0, 0)', 'important'); }
This code will work no matter how many inputs you've added to the slide. You just need to know how to properly target the input that needs to be disabled. Check the attached video demonstrating scripts above.
To target a unique ID of an input element, you might use something like id="acc-5nkaJ2cbCf9". To find and reference this ID in your script, you would first need to get it from the developer console and then update your script with a line such as document.getElementById('acc-5nkaJ2cbCf9'). If you need to use the same script on a different slide or with a different TEF, you would need to repeat this process to update the code. Now, imagine you have multiple TEFs that you want to enable or disable based on certain actions. This approach would not be reusable without constantly updating the code. Getting all inputs at the same time and then isolating the specific one you need by its index number proves to be the easiest method that works.
We can also make this more efficient by creating global functions to avoid repeating code, and run this code only when the timeline starts on this slide.
Now we can call a function to disable the TEF under certain conditions: window.disableInput(0); // 0,1,2,3...
Or call a function to enable the TEF under different conditions or when something else happens: window.enableInput(0); // 0,1,2,3...
37 Replies
Bumping this thread to add another reason for disabling text entry fields:
When reviewing a quiz question, a user can still tab through the focus order and change their answer, even with an object blocking the fields. Then when they return to the results slide it will mark that question as correct.
You can disable a text entry field at any time with just a few lines of JavaScript. Share your scenario or setup, and we'll make it work if feasible.
I can't share the specific file since it contains proprietary information but would you be able to share the lines of Javascript that are needed?
Thank you for your suggestion, but unfortunately this technique doesn't work when building a drop-down menu system that's embedded within a scroll panel. The layers are unable to track the location of the object within the scroll panel ... a layer simply shows up on top of whatever the existing content is.
I attached the .story file. The JavaScript code is inside Execute JavaScript triggers. Use it however and whenever you need. In this .story, I show how to simply enable or disable the text entry field with one button based on its state. In reply to Griffin Davis.
Thank you, Nadim! Works like a charm.
No problem, Rick. Glad it worked.
Thanks for that Nedim. I have many text entry fields on one slide. How would I tell the Javascipt to disable a specific one?
I tried to change it to reference the text entry field using accessibility text, but it's not working for me.
You should change selector querySelector('.acc-textinput') to querySelectorAll('.acc-textinput'). It selects all elements/inputs that match a specified CSS selector and returns a NodeList containing those elements. For example:
var inputs = document.querySelectorAll('.acc-textinput');
if (inputs.length > 0) {
inputs[1].disabled = true;
inputs[1].style.setProperty('color', '#BFBFBF', 'important');
}
It means that only the second element/input (input[0] being the first input) will be disabled. And enabled again with the code below:
var inputs = document.querySelectorAll('.acc-textinput');
if (inputs.length > 0) {
inputs[1].disabled = false;
inputs[1].style.setProperty('color', 'rgba(0, 0, 0, 0)', 'important');
}
This code will work no matter how many inputs you've added to the slide. You just need to know how to properly target the input that needs to be disabled. Check the attached video demonstrating scripts above.
That's super helpful Nedim; it works like a charm. Thanks so much!
Is the reason you did this based on the input numbers instead of a unique ID for each input just ease of coding?
To target a unique ID of an input element, you might use something like
id="acc-5nkaJ2cbCf9"
. To find and reference this ID in your script, you would first need to get it from the developer console and then update your script with a line such asdocument.getElementById('acc-5nkaJ2cbCf9')
. If you need to use the same script on a different slide or with a different TEF, you would need to repeat this process to update the code. Now, imagine you have multiple TEFs that you want to enable or disable based on certain actions. This approach would not be reusable without constantly updating the code. Getting all inputs at the same time and then isolating the specific one you need by its index number proves to be the easiest method that works.We can also make this more efficient by creating global functions to avoid repeating code, and run this code only when the timeline starts on this slide.
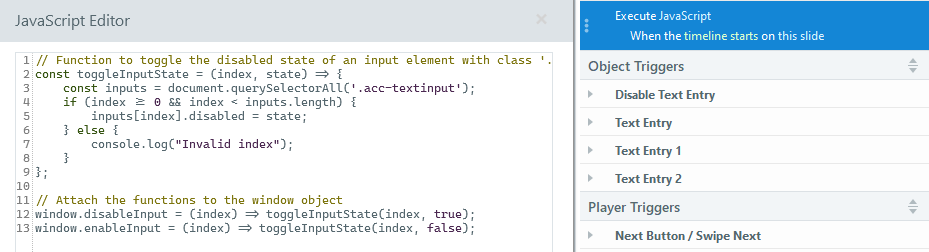

Now we can call a function to disable the TEF under certain conditions:
window.disableInput(0); // 0,1,2,3...
Or call a function to enable the TEF under different conditions or when something else happens: window.enableInput(0); // 0,1,2,3...